Strivacity’s Journey-flow API for Native Clients allows a brand’s developers to leverage all of the power of Strivacity login and registration flows inside of their native mobile and web applications. Brands can achieve this while their developers maintain full control of the presentation layer code. Strivacity provides SDKs to cover enable developers to implement into their native applications.
Benefits
Our native app sdk approach ensures the full capabilities of Strivacity are available to native clients. This includes full support for:
- Policy-defined workflows
- Custom Journey-builder workflows
- Identity Verification
- A/B Testing
- Lifecycle Event Hooks
- Any step or flow our hosted components can display
Our SDK approach allows brands to make cloud-side policy changes that are reflected in the native app without having to update any native app code. Essentially, brands can change the entire login or registration experience without their developers having to make any code changes or wait till users adopt a new version. This comes in handy in many situations, for example when performing A/B testing where different users get different experiences. Brand managers can rapidly iterate through experiments and experience changes without having to involve their native app development team.
Further, if the native app encounters any UI it cannot render from the API call, the SDK will fail-over to Strivacity no-code components to handle that step of the workflow. This gives brands the ability to add new features in an agile fashion.
Approach
Strivacity’s approach provides access to our policy-driven login and registration flows via these native SDKs. The process works like this:
- A native application kicks off an OIDC login flow via a function call in the SDK. The SDK then makes a REST API call to the Strivacity Journey-flow API to start the login flow.
- The Strivacity returns a JSON payload to the app that describes what UI widgets to render in the UI (e.g. logo, email address input field, and a continue button), and what information the app needs to return (e.g. email address)
- The app then interprets the payload, renders the UI, collects the information and returns any needed data via the SDK
- If the native application cannot render the UI described in the payload, it will fail over to hosted components
- The SDK will ask for a web view from the Strivacity server and
- Fail over to Strivacity no-code components instead of the native app rendering that step
As long as the native app knows how to render the needed widgets for any given step, the app can handle various combinations of screen definitions that may be passed to it by Strivacity. If a brand admin changes a policy for that app, the app will either render it with the proper experience or fail over to Strivacity no-code components to finish the task.
How to configure in Strivacity
The Journey Flow API is implemented by adding the client for OIDC using the Journey Flow API to an existing application.
To configure in the Strivacity admin console:
-
Go to Applications > (your applicaion) > Clients > Create client
-
Selec the "OIDC using the Journey Flow API" client type
-
Configure the client according to your needs (see configuring Clients for more informaiton)
-
Be sure to include a callback URL in the client configuration to point back to the application
-
This client is already configured to support the OIDC PKCE flow, which is required to use this API.
Once that client has been configured and saved, you are ready to begin incorporating it into your native application.
How to use the API
Initialize an OIDC flow
Strivacity's Journey-vlow API begins with an OIDC PKCE flow.
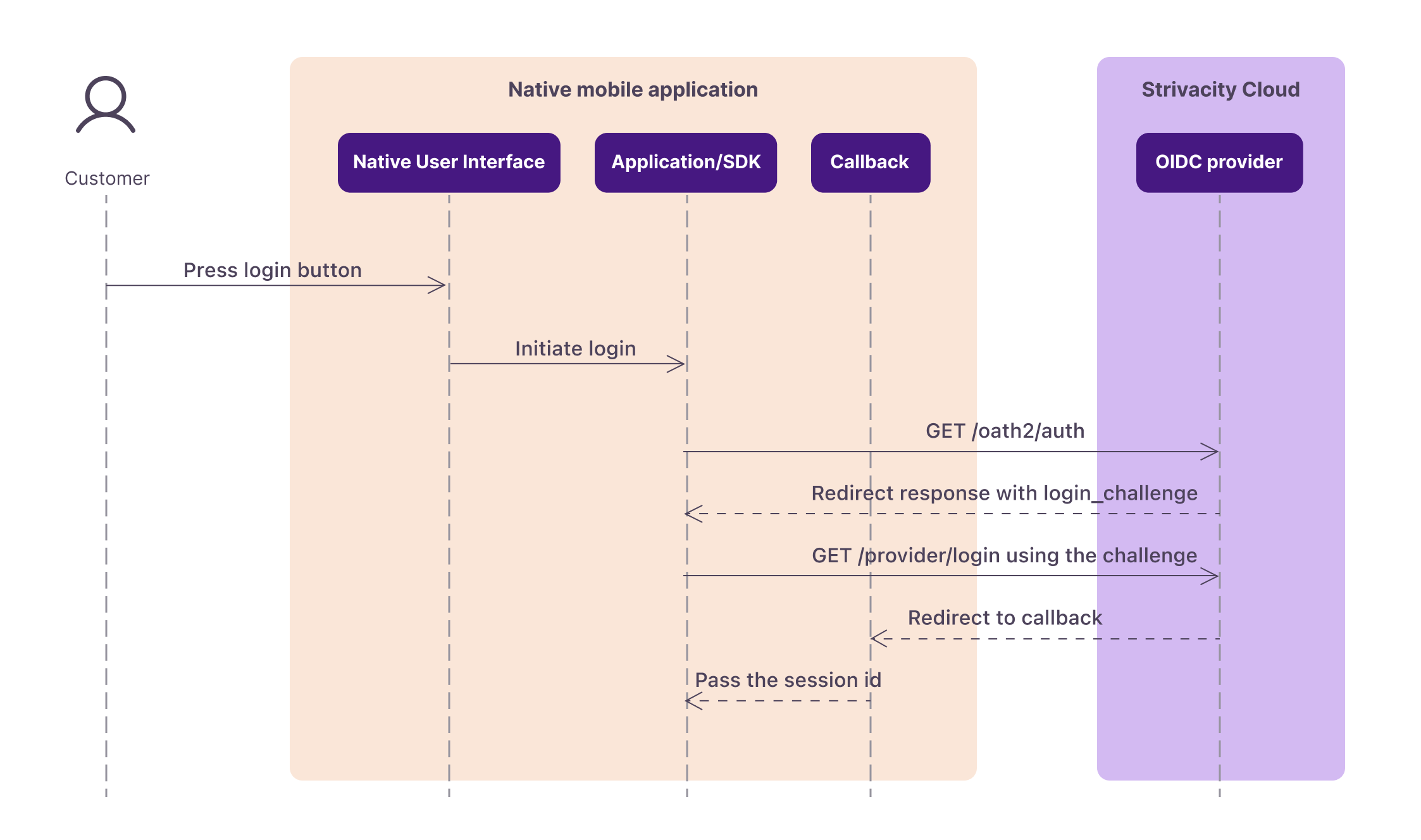
GET /auth
GET https://{{tenant}}/oauth2/auth
?client_id={{clientId}}
&redirect_uri={{redirectUrl}}
&scope=openid
&response_type=code
&response_mode=query
&code_challenge_method=S256
&code_challenge={{code_challenge}}
&state={{state}}
&nonce={{nonce}}
> {%
client.global.set("loginProvider", response.headers.valueOf('Location'))
%}
GET /provider/login
GET {{loginProvider}}
> {%
let nativeUrl = response.headers.valueOf('Location');
client.global.set("nativeUrl", nativeUrl)
client.global.set('sessionId', new URLSearchParams(nativeUrl.substring(nativeUrl.indexOf('?') + 1)).get('session_id'))
%}
Login and registration flows - native user interface
There are two options for implementing the Strivacity SDKs: Using native interfaces that are served by the native application or using a web view to display Strivacity no-code components.
Native user interface flow
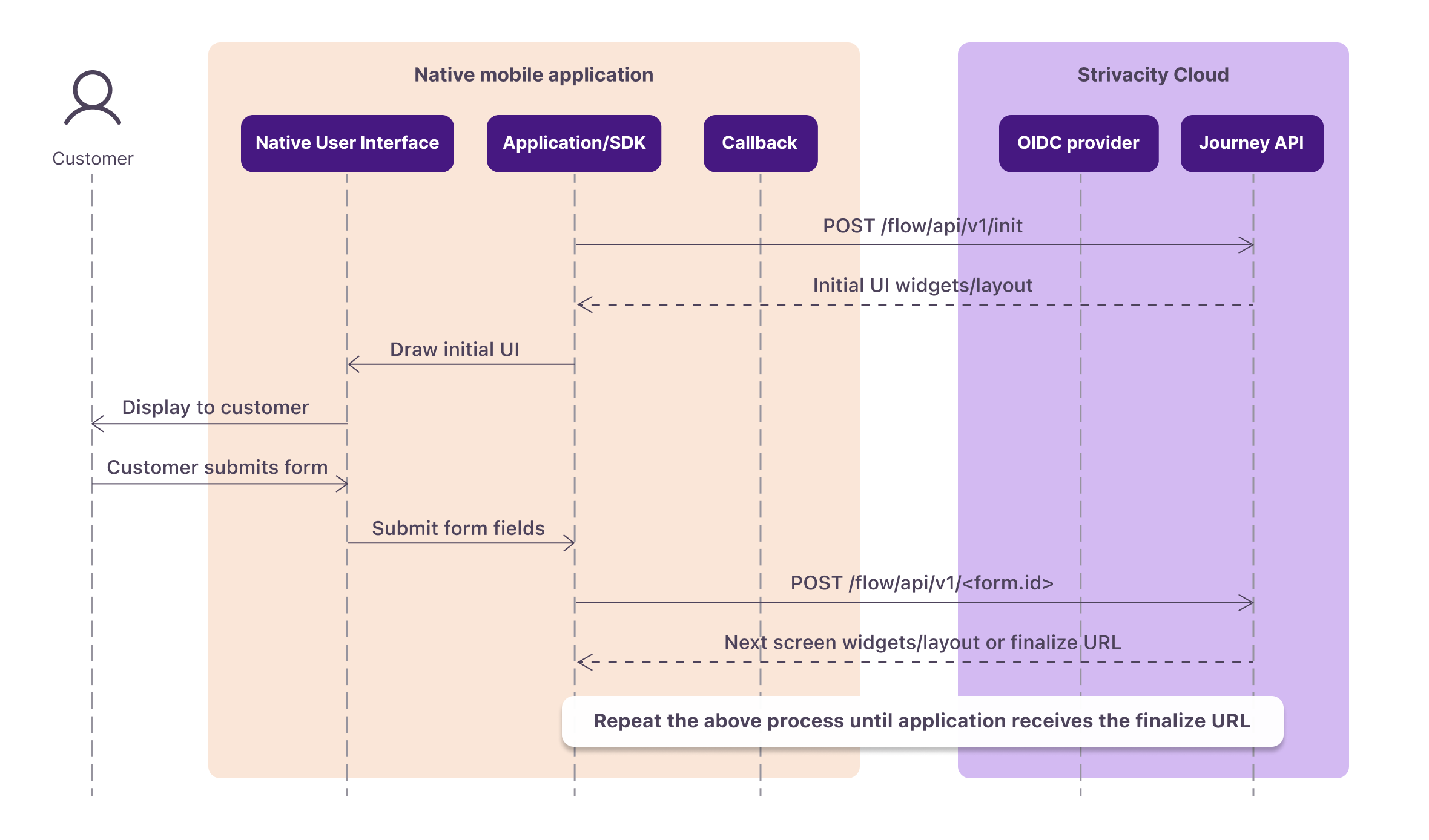
POST /flow/api/v1/init
POST https://{{tenant}}/flow/api/v1/init
Authorization: Bearer {{sessionId}}
> {%
console.log('init response: ' + JSON.stringify(response.body, null, 4))
console.log('Fallback url: ' + response.body.hostedUrl)
%}
POST /flow/api/v1/form/identifier
POST https://{{tenant}}/flow/api/v1/form/identifier
Authorization: Bearer {{sessionId}}
Content-Type: application/json
{
"identifier": "[email protected]"
}
> {%
console.log('identifier response: ' + JSON.stringify(response.body, null, 4))
%}
POST /flow/api/v1/form/password
POST https://{{tenant}}/flow/api/v1/form/password
Authorization: Bearer {{sessionId}}
Content-Type: application/json
{
"password": "{{password]]""
}
> {%
console.log('password response: ' + JSON.stringify(response.body, null, 4))
client.global.set("finalizeUrl", response.body.finalizeUrl)
%}
GET auth post login
GET {{finalizeUrl}}
> {%
client.global.set("consentProvider", response.headers.valueOf('Location'))
%}
GET consent provider
GET {{consentProvider}}
> {%
client.global.set("hydraFinish", response.headers.valueOf('Location'))
%}
GET auth post consent
GET {{hydraFinish}}
> {%
console.log('result: ' + response.headers.valueOf('Location'))
let callbackUrl = response.headers.valueOf('Location');
client.global.set('codeToken', new URLSearchParams(callbackUrl.substring(callbackUrl.indexOf('?') + 1)).get('code'))
%}
POST token endpoint
POST https://{{tenant}}/oauth2/token
Content-Type: application/x-www-form-urlencoded
grant_type=authorization_code
&client_id={{clientId}}
&code_verifier=VC9aHmX1Oe8C3jeAAmBVKvFTfugtt1rKFXoCmNOKBJc
&code={{codeToken}}
&redirect_uri={{redirectUrl}}
> {%
console.log('result: ' + JSON.stringify(response.body, null, 4))
%}
Falling back to no-code components
For brands who prefer to use no-code components rather than building their own native experiences, or when the native application encounters any functionality it doesn't understand, it can redirect to the fallback URL provided in the response to start or finish the flow using no-code componets instead of native user interfaces.
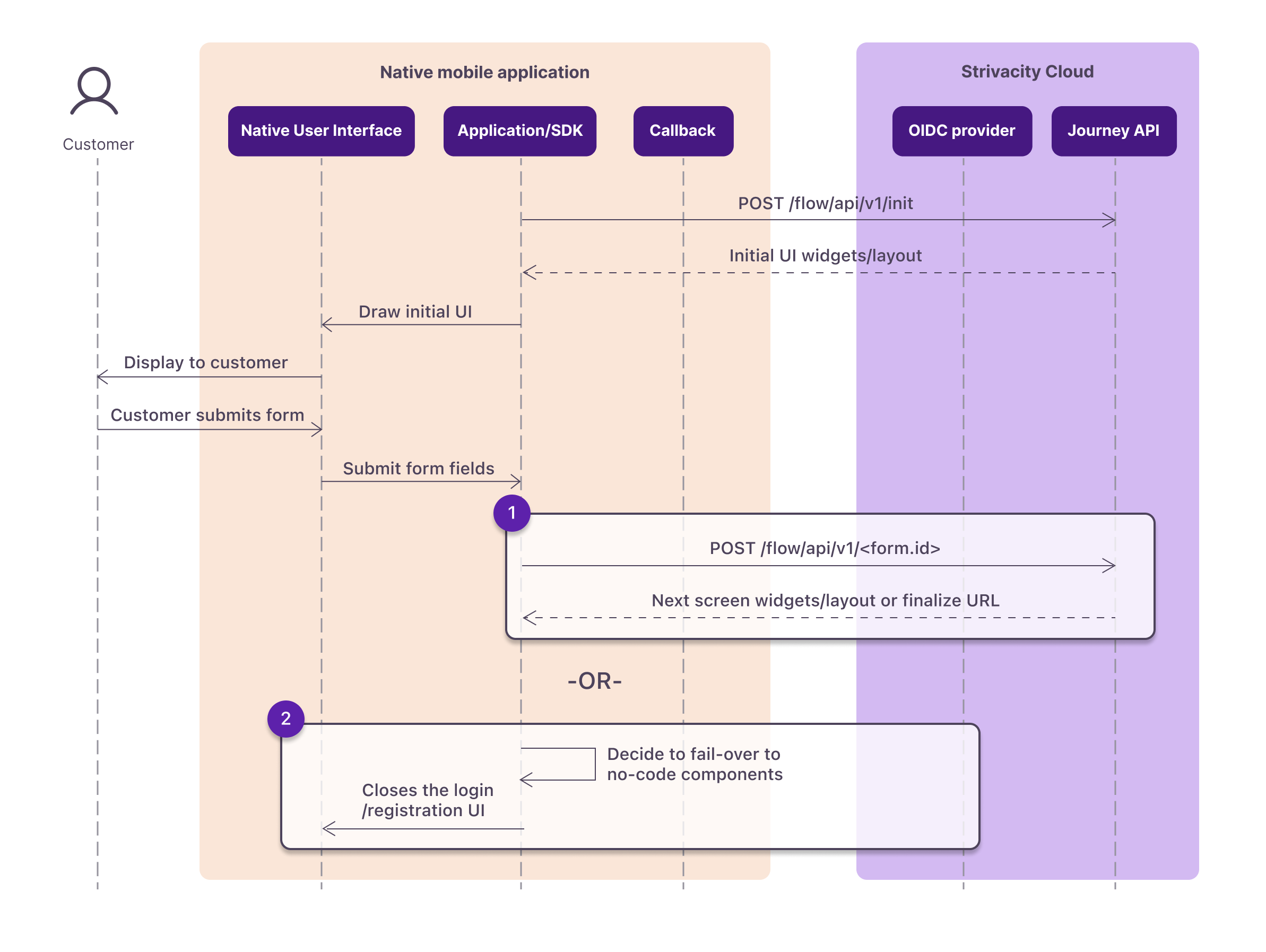
No-code components flow
In this flow, the web view in the native application displays no-code components to the customer who interacts with the hosted login until the flow is complete and a code token is returned.
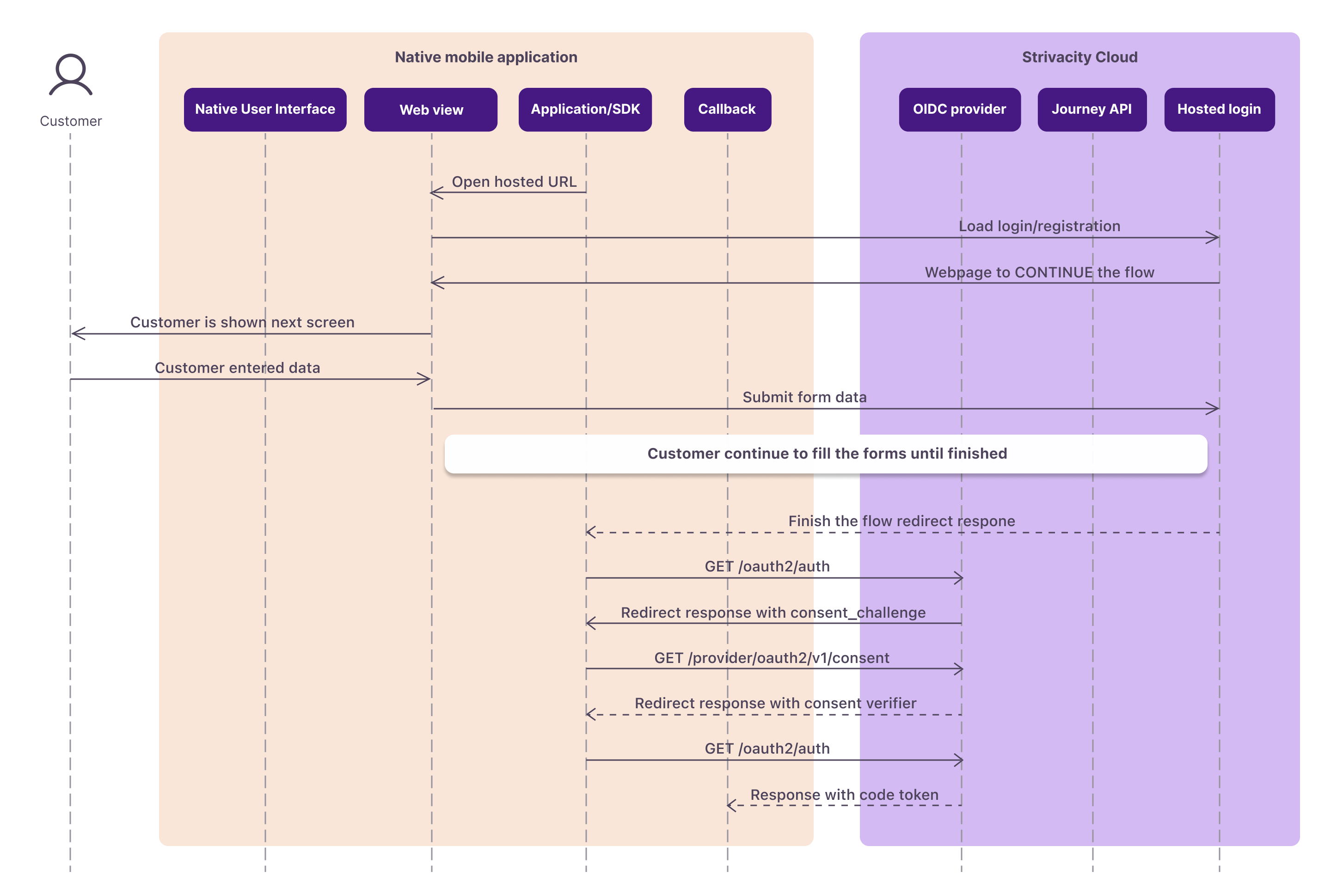